Ever heard the term ‘Ruby’ tossed around in tech discussions or job descriptions and wondered exactly what it means? You’re in the perfect place! In simple terms, Ruby is a popular and versatile programming language celebrated for its elegant, human-friendly syntax that often reads like plain English. It was uniquely designed with programmer happiness and productivity at its core. This guide will break down precisely what Ruby is, explore its standout features, uncover its most common applications (including its famous partner, Ruby on Rails!), and help you understand its place in the modern tech landscape. Let’s explore the world of Ruby together!
What Exactly is Ruby?
Ruby is an open-source, dynamic, general-purpose programming language focused on simplicity and productivity. It combines a syntax inspired by languages like Perl with object-oriented features influenced by Smalltalk. Think of it as a powerful tool for communicating instructions to computers, but designed to feel intuitive and expressive for the humans writing those instructions.
Ruby is considered a “high-level” language. This means its syntax is closer to human language and further away from the complex machine code that computers directly understand. This abstraction makes it easier for developers to write, read, and maintain code. It’s also “general-purpose,” indicating it’s not restricted to just one type of task; it can be used for web development, scripting, data processing, and more.
The story of Ruby began in the mid-1990s with Japanese computer scientist Yukihiro Matsumoto, often known simply as “Matz”. He wasn’t satisfied with existing scripting languages like Perl or Python at the time. Matz sought to create a language that blended the functional aspects he liked from some languages with a truly consistent object-oriented approach, all while prioritizing “developer happiness.” His goal was to make programming enjoyable and efficient, leading to a language renowned for its elegant design.
This philosophy permeates Ruby. It aims for a balance, providing powerful capabilities without overwhelming complexity. It often provides multiple ways to achieve the same task, allowing developers to choose the most expressive method for their specific situation.
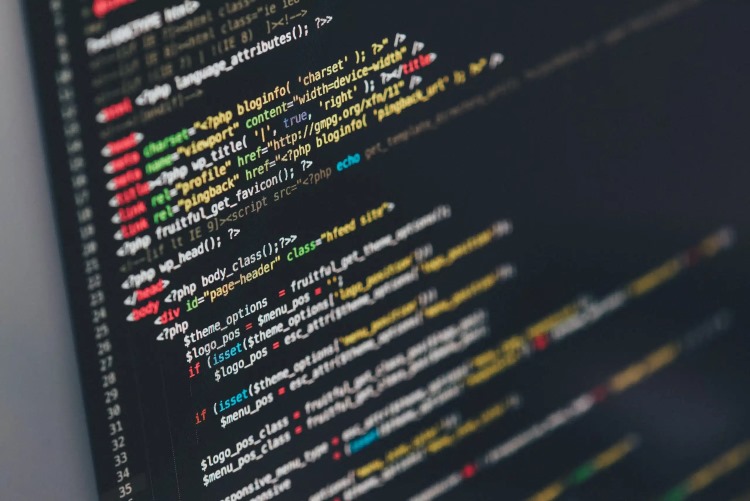
Key Features: What Makes Ruby Stand Out?
Ruby possesses several core characteristics that define its identity and contribute to its appeal among developers. These features influence how Ruby code is written and executed.
Truly Object-Oriented (Everything is an Object)
In Ruby, fundamentally everything is treated as an object, meaning data and the functions (methods) that operate on it are conceptually bundled together. Even basic data types like numbers (e.g., 5
) or text (“hello”) are objects and have built-in capabilities (methods) you can use directly.
An “object” in programming is like a self-contained unit. Imagine a real-world object like a Car
. It has properties (attributes) like color
, make
, model
, and it has actions it can perform (methods) like start_engine()
, accelerate()
, brake()
. In Ruby, you can define your own objects (like a Car
class) to model real-world or abstract concepts in your code. This approach encourages modularity (breaking code into manageable pieces) and reusability (using the same object definition multiple times). For example, the simple expression 5.times { ... }
works because 5
is an object with a method called times
.
Interpreted Language (No Pre-Compilation Needed)
Ruby is an interpreted language, meaning Ruby code is executed line-by-line directly by another program called an interpreter at runtime. This differs from compiled languages like C++ or Java, where the entire source code must first be translated into low-level machine code by a compiler before the program can run.
When you run a Ruby script, the Ruby interpreter reads the code and executes its instructions sequentially. This generally leads to a faster development cycle: you can write code, run it immediately to test, make changes, and run again without waiting for a compilation step. This makes Ruby well-suited for scripting (automating tasks) and web development where rapid iteration is often beneficial. It also enhances portability; Ruby code can often run on any system (Windows, macOS, Linux) that has the appropriate Ruby interpreter installed.
Dynamic Typing (Flexibility in Variables)
Ruby employs dynamic typing, meaning the type of data a variable holds (e.g., number, text, list) is checked during program execution (runtime), not declared explicitly by the programmer beforehand. You can create a variable and assign it a number, and later assign it a string of text without issue.
Let’s clarify “variable type”: it refers to the kind of data being stored, such as an integer (whole number), a float (decimal number), a string (text), or a boolean (true/false). In statically-typed languages like Java or C#, you must declare the type: int age = 30;
. In Ruby, you simply write age = 30
, and Ruby figures out age
holds an integer. If you later write age = "thirty"
, Ruby adapts.
This dynamic nature offers flexibility and can speed up initial code writing. However, it also means type-related errors might only surface when the program runs, rather than being caught during a compilation phase. This underscores the importance of thorough testing in dynamically typed languages.
Elegant and Readable Syntax
Ruby is consistently praised for its clean, elegant syntax that often resembles natural English, enhancing code readability and maintainability. This was a core design goal set by Matz, aiming to reduce the “cognitive load” on developers.
Ruby syntax often minimizes the need for punctuation like semicolons at the end of lines or excessive parentheses. It favors using English words for control structures (like if
, unless
, while
) and method calls. For instance, to print “Ruby!” five times, you can intuitively write:
5.times do
print "Ruby! "
end
# Output: Ruby! Ruby! Ruby! Ruby! Ruby!
Here, 5
is an object, times
is a method called on that object, and the code block { ... }
or do ... end
specifies what action to perform each time. This readability makes it easier for developers (including those new to the code) to understand what’s happening.
Automatic Memory Management (Garbage Collection)
Ruby automatically manages computer memory using a process called garbage collection (GC), relieving developers from the complex task of manual memory allocation and deallocation. In lower-level languages, programmers often need to explicitly request memory to store data and then release it when it’s no longer needed – errors here can lead to bugs or crashes.
Ruby’s garbage collector periodically runs in the background, identifying memory occupied by objects that are no longer being used by the program (the “garbage”). It then reclaims this memory, making it available for future use. This significantly simplifies development and helps prevent common memory-related errors like memory leaks, allowing developers to focus more on application logic.
What is Ruby Used For? Common Applications
While Ruby is a general-purpose language, its features and ecosystem make it particularly well-suited for certain tasks. Its use cases have evolved, but it remains strong in several key areas.
Powering Web Development (Especially with Ruby on Rails)
Ruby’s most significant and widespread application is in web development, primarily driven by the highly influential Ruby on Rails framework. Ruby on Rails, often shortened to “Rails,” is a comprehensive framework built using Ruby that drastically simplifies the creation of complex, database-backed web applications.
Rails provides developers with a structured approach (using the MVC – Model-View-Controller pattern) and a vast set of tools and conventions for handling common web development tasks. MVC is an architectural pattern that separates application logic into three interconnected parts: the Model (manages data and business logic), the View (handles the user interface and presentation), and the Controller (takes user input and interacts with the Model and View). Rails follows principles like “Convention over Configuration” and “Don’t Repeat Yourself” (DRY) to streamline development.
Many well-known websites and applications have used Ruby on Rails, especially during their startup phases, including platforms like GitHub, Airbnb, Shopify (initial versions), and Basecamp. Ruby (via Rails) is predominantly used for backend development, handling the server-side logic, database interactions, user authentication, and API (Application Programming Interface) creation that power web applications.
Rapid Prototyping
Ruby’s concise syntax combined with the productivity boosts from frameworks like Rails makes it an excellent choice for rapidly developing prototypes and Minimum Viable Products (MVPs). An MVP is a version of a new product with just enough features to be usable by early customers who can then provide feedback for future product development.
Startups and development teams often leverage Ruby/Rails to quickly build and test ideas, get functional applications up and running efficiently, and iterate based on user feedback without getting bogged down in boilerplate code.
Automation, Scripting, and Command-Line Tools
Ruby functions effectively as a scripting language for automating repetitive tasks, managing systems, and building custom command-line interface (CLI) tools. A script is typically a shorter program designed to automate a sequence of operations.
Its readable syntax and strong text manipulation capabilities make it suitable for writing scripts that handle tasks like file processing, system administration, running automated tests, or creating build tools (like the popular Ruby tool Rake) and deployment tools (like Capistrano).
Data Processing and Analysis (Basic to Intermediate)
While Python currently dominates the specialized field of data science, Ruby possesses capabilities for basic-to-intermediate data processing, file manipulation, and analysis tasks. There are Ruby libraries (“gems,” explained later) available for working with data formats like CSV and JSON, interacting with databases, and performing calculations.
For heavy-duty numerical computing, machine learning, or large-scale data analysis, languages like Python with their extensive specialized libraries (e.g., NumPy, Pandas, Scikit-learn) are generally preferred. However, Ruby can certainly handle less complex data wrangling and processing needs effectively.
Ruby vs. Ruby on Rails: Understanding the Difference
It’s essential to distinguish clearly: Ruby is the programming language itself, while Ruby on Rails (or simply Rails) is a software framework built using the Ruby language. This is a common point of confusion for beginners.
Think of it like this:
- Ruby is the English language – it provides the vocabulary, grammar, and syntax rules.
- Ruby on Rails is like a highly structured template or software suite for writing a specific type of document, like a novel or a business report, using the English language. The template provides chapter outlines, formatting tools, character tracking features, etc., making the writing process faster and more organized for that specific task.
Rails provides a full-stack framework specifically designed for building web applications. It leverages Ruby’s syntax and features but adds a vast amount of pre-written code, structure (like the MVC pattern mentioned earlier), and conventions to handle common web tasks like routing requests, interacting with databases, and rendering HTML pages. You write Ruby code within the structure provided by the Rails framework. You cannot use Rails without Ruby, but you can use Ruby for many things without Rails.
Is Ruby Still Relevant in 2025?
Yes, as of April 11, 2025, Ruby remains a relevant and actively used programming language, particularly within the web development sphere powered by Ruby on Rails. While the landscape of programming languages is constantly evolving, with languages like JavaScript (Node.js) and Python (Django/Flask) gaining significant popularity for web development, Ruby has carved out a stable and enduring niche.
Ruby and Rails are known for their maturity and stability. Many large, successful companies continue to run and maintain significant applications built with Rails (e.g., GitHub, Shopify, Airbnb). The focus on developer productivity and code readability makes it attractive for teams building complex applications and for startups needing to move quickly.
Furthermore, Ruby boasts a strong and vibrant community and ecosystem. RubyGems is the official package manager for Ruby, hosting hundreds of thousands of reusable libraries (called “gems”) that developers can easily incorporate into their projects to add functionality without writing it from scratch. This ecosystem covers everything from database connectors to testing frameworks to API clients. Active online communities, forums, and conferences ensure ongoing support and development for the language and its related tools.
While the overall number of Ruby/Rails job postings might be smaller compared to JavaScript or Python in some regions, demand persists, especially for developers experienced with maintaining and extending existing Rails applications or for companies that value the specific productivity benefits of the framework.
Should You Consider Learning Ruby?
Deciding whether to learn Ruby depends largely on your individual goals and interests within the tech field.
Who is Ruby Good For?
Ruby is often a good choice for aspiring web developers (especially those interested in backend development with Rails), beginners seeking a highly readable first programming language, and development teams prioritizing rapid development cycles. Its gentle learning curve for basic concepts and its emphasis on clear code can make it less intimidating for newcomers compared to some other languages.
Potential Advantages
Key advantages of learning and using Ruby include its exceptionally readable and elegant syntax, the powerful and convention-driven Ruby on Rails framework for web development, and a core philosophy centered on developer happiness and productivity. The extensive library collection via RubyGems and the supportive community are also significant benefits. Working with Ruby, especially within Rails, often feels efficient and allows developers to focus on building features rather than boilerplate code.
Things to Consider
Important considerations include Ruby’s runtime performance, which can be slower than compiled languages (like Go or Java) or even Node.js for certain high-concurrency, I/O-bound tasks. While performance has improved significantly over the years (with advancements in Ruby interpreters like YJIT), it might not be the optimal choice for applications where raw execution speed is the absolute top priority. Additionally, while demand exists, the overall job market for Ruby might be considered more niche compared to the vast markets for JavaScript or Python developers.
How to Get Started with Ruby (Your Next Steps)
Getting started with Ruby typically involves installing the language interpreter on your computer, exploring interactive coding environments, and leveraging the wealth of online learning resources.
- Install Ruby: Visit the official Ruby language website at ruby-lang.org. It provides instructions and downloads for installing Ruby on Windows, macOS, and Linux. Version managers like
rbenv
orRVM
are often recommended for managing multiple Ruby versions easily. - Use Interactive Ruby (IRB): Once installed, you can type
irb
in your terminal or command prompt. This opens Interactive Ruby, a tool (a REPL: Read-Eval-Print Loop) where you can type Ruby code snippets and see the results immediately. It’s an excellent way to experiment and learn basic syntax. - Explore Online Tutorials & Courses: Numerous websites offer free and paid resources for learning Ruby, ranging from beginner tutorials to in-depth courses. Platforms like Codecademy, freeCodeCamp, The Odin Project, and Coursera often have Ruby tracks. Look for resources specifically labeled “Ruby tutorial for beginners.”
- Read Documentation: The official Ruby documentation on ruby-lang.org is a comprehensive resource, though it can be dense for absolute beginners. It’s invaluable once you have the basics down.
- Practice: Like any skill, programming requires practice. Try solving small coding challenges, building simple scripts, or working through a beginner-friendly Ruby on Rails tutorial once you’re comfortable with the core Ruby language.
Wrapping Up: Your Ruby Takeaways
In summary, Ruby is an elegant, dynamically typed, purely object-oriented programming language specifically designed with developer productivity and happiness in mind. While versatile, it is most famous for its role in web development, thanks largely to the powerful and popular Ruby on Rails framework.
Remember its key characteristics: interpreted execution, dynamic typing for flexibility, a remarkably readable syntax, and automatic memory management via garbage collection. Though the tech landscape evolves, Ruby maintains its relevance through a mature ecosystem, a dedicated community, and its continued success in powering countless web applications. Whether you’re a complete beginner or an experienced developer exploring new tools, Ruby offers a unique and often rewarding programming experience.