If you’ve ever wondered how websites do more than just show static text and images – how they move, update, and interact with you – the answer is often JavaScript. So, what is JavaScript? In simple terms, it’s a powerful programming language that breathes life into the web. It’s a fundamental tool for developers everywhere, transforming static pages into dynamic and engaging experiences. In this guide for beginners, we’ll break down exactly what JavaScript is, what it’s used for, and why it’s an essential part of the internet as you know it.
Understanding the Basics of JavaScript
What Exactly Is JavaScript?
At its core, JavaScript is a programming language that runs in web browsers, enabling dynamic behavior on websites. Think of HTML as the skeleton of a webpage and CSS as its clothes; JavaScript is the muscle that makes it move and respond. It allows elements on a page to change, update, and react to user input without requiring the entire page to reload.
JavaScript is categorized as a scripting language, meaning its code is typically executed line by line. It empowers developers to control the behavior of web pages after they have been loaded in the browser. This includes everything from simple animations and interactive forms to complex single-page applications that feel more like desktop software.
Unlike compiled languages where code is translated into machine code before execution, JavaScript is often interpreted directly by the browser’s JavaScript engine. However, modern engines employ techniques like Just-In-Time (JIT) compilation to optimize performance, making JavaScript execution remarkably fast.
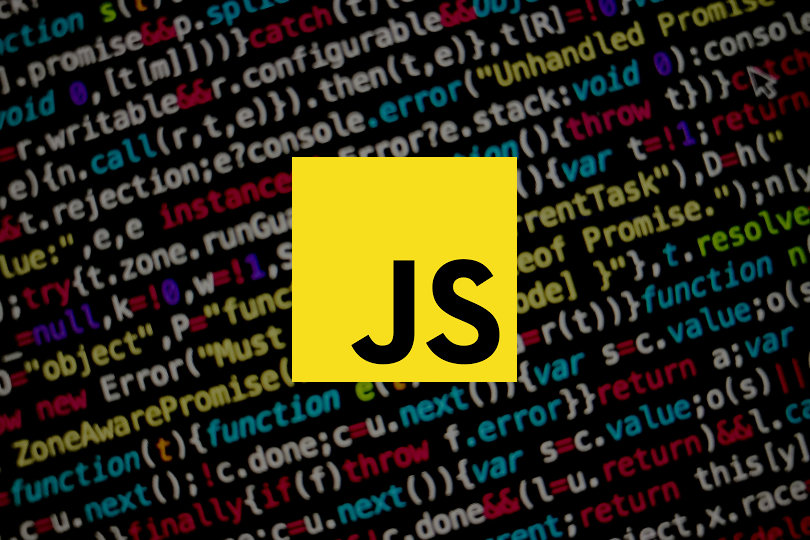
Why is JavaScript So Important?
JavaScript’s importance stems from its ability to significantly enhance the user experience on the web. It allows for dynamic content updates, such as displaying real-time information, creating interactive maps, and implementing complex user interfaces that respond instantly to actions like clicks and hovers.
Executing code on the client-side (in the user’s browser) with JavaScript improves website responsiveness. Instead of constantly sending requests to the server for every interaction, JavaScript can handle many tasks directly in the browser, leading to faster load times and a smoother, more engaging experience for the user.
Beyond the front-end, JavaScript’s versatility has expanded significantly with the advent of Node.js. This runtime environment allows JavaScript to be used for server-side programming, enabling the development of full-stack web applications using a single language. This unified approach streamlines development workflows.
Furthermore, JavaScript boasts an incredibly large and active community of developers worldwide. This vast community contributes to a rich ecosystem of libraries, frameworks, and tools, making it easier and faster to build complex applications and find solutions to development challenges.
How does JavaScript Works?
Client-Side vs. Server-Side JavaScript
In the context of web development, JavaScript primarily operates on the client-side, meaning the code is executed by the user’s web browser. When a user visits a webpage, the browser downloads the HTML, CSS, and JavaScript files. The browser’s JavaScript engine then interprets and runs the JavaScript code, bringing the page to life.
However, the introduction of Node.js revolutionized JavaScript by enabling its use on the server-side. Node.js provides a JavaScript runtime environment that can execute JavaScript code outside of a web browser. This allows developers to build backend logic, handle databases, and create APIs (Application Programming Interfaces) using JavaScript.
For beginners, understanding client-side JavaScript is crucial as it directly impacts the interactivity and user interface of websites they see every day. Server-side JavaScript with Node.js is a more advanced topic but highlights the language’s growing versatility across the entire web development stack.
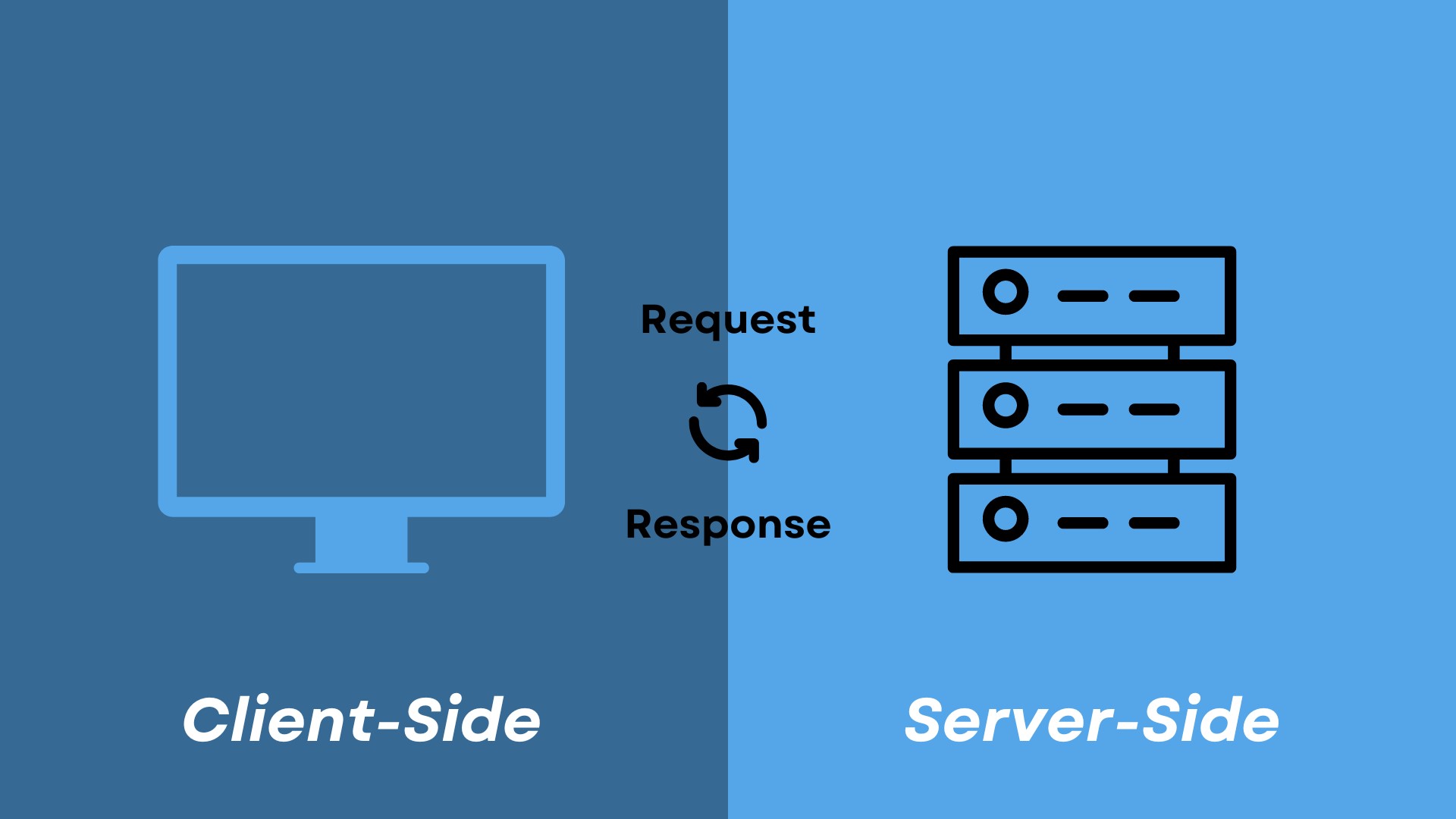
Key Concepts in JavaScript
To grasp how JavaScript works, it’s essential to understand some fundamental concepts. Variables are like containers that hold information, such as text, numbers, or even more complex data. You can think of them as labeled boxes where you can store and retrieve values as needed throughout your code.
Data types classify the different kinds of values that variables can hold. Common data types include numbers (e.g., 10, 3.14), strings (text, like “Hello”), booleans (true or false), and more complex structures like objects and arrays, which allow you to group and organize related data.
Operators are symbols that perform actions on values. For example, the +
operator can add numbers or concatenate strings, the -
operator subtracts numbers, and the ===
operator checks if two values are exactly equal. Operators are fundamental for performing calculations and making comparisons in your code.
Functions are reusable blocks of code that perform specific tasks. You can define a function once and then call it multiple times with different inputs to achieve the same operation without rewriting the code each time. Functions help organize code and make it more modular and maintainable.
Events are actions that occur in the browser, often as a result of user interaction. Examples include a user clicking a button, moving their mouse over an element, or the page finishing loading. JavaScript can “listen” for these events and execute specific code in response, making websites interactive.
The DOM (Document Object Model) is a programming interface for web documents. It represents the structure of an HTML or XML document as a tree-like structure, where each element, attribute, and piece of text is a node in the tree. JavaScript can interact with the DOM to dynamically change the content, structure, and style of a webpage. For instance, it can add new elements, remove existing ones, modify text, or change CSS styles in response to user actions or other events.
A Quick Look at JavaScript’s History
The Birth of JavaScript
JavaScript was created in a remarkably short period – just ten days in May 1995 by Brendan Eich, who was working at Netscape Communications Corporation (now Mozilla Corporation). Initially, it was developed under the name “Mocha,” and later briefly known as “LiveScript.”
The language was strategically renamed “JavaScript” to capitalize on the popularity of Java at the time, although the two languages have fundamentally different designs and purposes. JavaScript was intended to be a lightweight scripting language that could complement Java’s more robust capabilities within the browser.
The first browser to support JavaScript was Netscape Navigator 2.0, released in December 1995. This marked the beginning of JavaScript’s journey to becoming an indispensable part of the World Wide Web, enabling the first wave of truly interactive web experiences.
Standardization with ECMAScript
The rapid adoption of JavaScript led to the need for standardization to ensure interoperability across different browsers. In 1997, Netscape submitted JavaScript to ECMA International, a standards organization. This resulted in the creation of the ECMAScript standard, which serves as the official specification for JavaScript.
ECMAScript provides a common set of rules and guidelines for implementing JavaScript. While “JavaScript” is the more commonly used name, “ECMAScript” is the formal standard that various JavaScript engines (like V8 in Chrome and Node.js, SpiderMonkey in Firefox) adhere to. This standardization has been crucial for the consistent evolution and widespread adoption of the language.
The Evolution to Modern JavaScript
JavaScript has undergone significant evolution since its inception. Early versions had limitations, but subsequent updates have introduced powerful new features and paradigms. Key milestones include the release of ECMAScript 5 (ES5) in 2009, which brought improvements like strict mode and JSON support, and ECMAScript 6 (ES2015), also known as ES6 or ES2015, which introduced a wealth of modern features such as classes, modules, arrow functions, and more concise syntax.
The language continues to evolve with new ECMAScript specifications released annually, bringing further enhancements and capabilities. These ongoing improvements ensure that JavaScript remains a relevant and powerful language for modern web development and beyond.
What Can You Do With JavaScript?
Enhancing Website Interactivity
One of the primary uses of JavaScript is to add interactivity to websites. This can range from simple effects like making a button change color when you hover over it to more complex interactions such as dropdown menus, image carousels, and dynamic form validation that provides instant feedback to users as they fill out information.
For example, imagine a website with a contact form. Using JavaScript, you can implement real-time validation to check if the email address entered is in the correct format before the user submits the form. This provides immediate feedback and improves the user experience by preventing unnecessary page reloads and server-side error messages.
Another common example is creating interactive image galleries. JavaScript can be used to build features like thumbnail navigation, image zooming, and slideshows, allowing users to engage with visual content in a more dynamic way. Animations, such as elements fading in or sliding onto the screen, also contribute to a more polished and engaging user experience, often powered by JavaScript.
Building Dynamic User Interfaces
JavaScript is also fundamental in building dynamic user interfaces (UIs), especially for Single Page Applications (SPAs). SPAs are web applications that load a single HTML page and dynamically update the content as the user interacts with them, without requiring full page reloads. This results in a smoother, more app-like experience.
Popular JavaScript frameworks like React, Angular, and Vue.js are specifically designed for building complex and interactive UIs for SPAs. These frameworks provide structures and tools that help developers manage the complexity of large-scale applications with features like component-based architecture and efficient DOM updates.
Consider a modern social media platform. As you navigate between different sections (e.g., your feed, profile, messages), the page doesn’t fully reload each time. Instead, JavaScript frameworks handle updating only the necessary parts of the UI, providing a seamless and responsive user experience.
Powering Web Applications
Beyond just adding visual effects and UI elements, JavaScript plays a crucial role in the functionality of web applications. It handles tasks like making asynchronous requests to servers (using AJAX or Fetch API) to retrieve and update data without interrupting the user’s current activity. This is essential for features like live updates, chat applications, and collaborative editing tools.
For instance, when you use an online document editor like Google Docs, JavaScript is constantly communicating with the server in the background as you type, saving your changes and updating the document in real-time for collaborators. This seamless interaction is powered by JavaScript’s ability to handle asynchronous communication.
Furthermore, JavaScript is used to manipulate and process data on the client-side, reducing the load on the server and improving performance. Tasks like sorting, filtering, and displaying large datasets can often be efficiently handled within the browser using JavaScript.
Beyond the Browser
The versatility of JavaScript extends far beyond traditional web browsers. With the advent of Node.js, JavaScript can now be used for server-side programming. This allows developers to build entire web applications using a single language, from the front-end UI to the backend API and server logic.
Node.js has enabled the creation of scalable and high-performance web servers and backend services. Many popular tools and platforms, such as npm (Node Package Manager), have emerged within the Node.js ecosystem, further expanding JavaScript’s reach and capabilities.
Moreover, JavaScript is also used in mobile app development through frameworks like React Native and Ionic. These frameworks allow developers to write code once in JavaScript and deploy it on both iOS and Android platforms, facilitating cross-platform mobile development.
Additionally, JavaScript is finding applications in areas like desktop application development (with Electron), game development (with libraries like Phaser), and even in emerging fields like the Internet of Things (IoT) and machine learning (with TensorFlow.js). This broad applicability underscores the power and flexibility of the JavaScript language.
JavaScript vs. Other Technologies
JavaScript vs. HTML
HTML (HyperText Markup Language) is the standard markup language for creating the structure and content of web pages. It uses tags to define elements like headings, paragraphs, images, and links. Think of HTML as the blueprint of a house, defining where the walls, doors, and windows are located.
JavaScript, on the other hand, adds behavior and interactivity to these HTML structures. While HTML defines what elements are on a page, JavaScript determines how those elements behave and respond to user actions or other events. JavaScript can dynamically modify the HTML structure, change the content of elements, and alter their properties.
For example, HTML can create a button on a webpage. However, it’s JavaScript that enables that button to do something when a user clicks it, such as submitting a form, displaying a message, or triggering an animation. HTML provides the static foundation, while JavaScript brings it to life.
JavaScript vs. CSS
CSS (Cascading Style Sheets) is the language used to style the visual presentation of web pages. It controls aspects like colors, fonts, layout, and responsiveness. If HTML is the blueprint and JavaScript is the muscle, then CSS is the interior and exterior design, dictating how the house looks.
While JavaScript can also manipulate the styles of HTML elements, the primary role of CSS is for styling. JavaScript’s involvement in styling is usually to change styles dynamically in response to user interactions or application logic. For instance, JavaScript might add a CSS class to an element to change its appearance when a certain condition is met.
It’s important to understand the separation of concerns: HTML for structure, CSS for styling, and JavaScript for behavior. While they can interact, keeping their primary roles distinct leads to more organized and maintainable code.
JavaScript vs. Java
Despite the similarity in their names, JavaScript and Java are fundamentally different programming languages. Java is a more complex, statically-typed, compiled language that is often used for building large-scale enterprise applications, Android mobile apps (historically), and server-side applications.
JavaScript, as discussed, is primarily a dynamically-typed, interpreted (initially), and prototype-based scripting language that is essential for front-end web development and increasingly used on the server-side with Node.js. While both languages share some common syntax elements due to historical reasons, their core concepts, use cases, and ecosystems differ significantly.
For beginners in web development, JavaScript is the more relevant language to focus on for creating interactive front-end experiences. Java plays a different role in the broader software development landscape. The naming similarity was largely a marketing strategy during JavaScript’s early days to associate it with the then-popular Java.
Getting Started with JavaScript
What You Need to Begin
The great thing about learning JavaScript for web development is that you likely already have the basic tools you need. A modern web browser (like Chrome, Firefox, Safari, or Edge) has a built-in JavaScript engine that can execute JavaScript code. You don’t need to install any special software to run client-side JavaScript.
You will also need a text editor to write your JavaScript code. While simple text editors like Notepad (on Windows) or TextEdit (on macOS) can work for basic examples, more specialized code editors like Visual Studio Code (VS Code), Sublime Text, or Atom offer features like syntax highlighting, code completion, and debugging tools that can greatly enhance your development experience. Most of these code editors are free to use.
As you progress, you might explore more advanced development environments and tools, but for learning the basics of JavaScript, a browser and a good text editor are sufficient to get started.
Your First Snippet of JavaScript
Let’s look at a very simple example of JavaScript code. You can write this code in your text editor and save it as an HTML file (e.g., index.html
):
<!DOCTYPE html>
<html>
<head>
<title>My First JavaScript</title>
</head>
<body>
<h1>Hello, World!</h1>
<button onclick="alert('You clicked the button!')">Click Me</button>
<script>
console.log("This message will appear in the browser's console.");
</script>
</body>
</html>
In this example:
- The
<button>
element has anonclick
attribute. This is an example of an event handler. When the button is clicked, the JavaScript codealert('You clicked the button!')
is executed, which displays a pop-up message in the browser. - The
<script>
tags enclose JavaScript code that will be executed when the browser loads the page. In this case,console.log("This message will appear in the browser's console.");
will print the message to the browser’s developer console (which you can usually open by pressing F12).
This simple example demonstrates how JavaScript can add interactivity (the button click) and output information (to the console) on a webpage.
Where to Learn More
If you’re eager to delve deeper into JavaScript, numerous excellent resources are available for beginners. Reputable online platforms like:
- MDN Web Docs (Mozilla Developer Network): Offers comprehensive documentation, tutorials, and guides on JavaScript and web development technologies.
- freeCodeCamp: Provides free, interactive coding courses and projects, including extensive JavaScript curriculum.
- Codecademy: Offers interactive lessons that allow you to learn by doing.
- Udemy and Coursera: Host a wide range of JavaScript courses, from beginner to advanced levels, often taught by experienced instructors.
Additionally, exploring online communities like Stack Overflow can be invaluable for finding answers to specific questions and learning from other developers’ experiences. Remember to practice regularly and work on small projects to solidify your understanding of JavaScript concepts.
Conclusion
JavaScript is an incredibly powerful and indispensable language for modern web development. From adding simple interactivity to building complex single-page applications and even venturing into server-side and mobile development, its versatility is unmatched. Its large and active community ensures a wealth of resources and continuous evolution, making it a vital skill for anyone looking to understand or build for the web.
As you embark on your journey to learn more about JavaScript, remember that even the most complex applications are built upon fundamental concepts. By understanding the basics and gradually exploring its capabilities, you’ll unlock the power behind the dynamic and engaging websites we interact with every day. The world of web development is vast and exciting, and JavaScript is a key that opens many doors.