Ever wondered what makes a website look good and feel intuitive to use? That’s the magic of frontend development! If you’re new to the world of tech, exploring career options, or simply curious about how the websites you visit are built, you’re in the right place. This guide will break down exactly what frontend development is in simple terms. We’ll explore the essential tools like HTML, CSS, and JavaScript, clarify the crucial difference between frontend and backend, touch on what a frontend developer does, and show you why it’s such a vital part of today’s digital world.
What is Frontend Development?
Frontend development specifically deals with the client-side of a web application. This term refers to everything that runs or is displayed within the user’s web browser (like Chrome, Firefox, or Safari) on their computer, tablet, or smartphone. It’s the part you directly engage with.
Think about any website you visit – the text you read, the images you see, the buttons you click, the forms you fill out. All these elements are part of the frontend. They are rendered and managed by your browser using code sent from the website’s server.
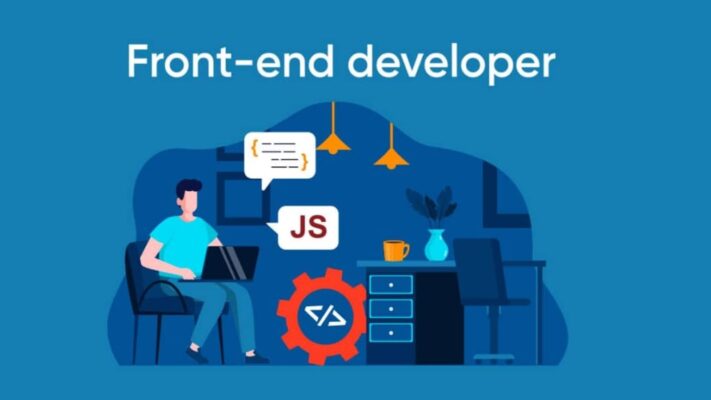
Defining the “Client-Side”
The “client” in client-side development is simply the user’s web browser or device accessing the website. Frontend code (HTML, CSS, JavaScript) is downloaded from the server and then executed or interpreted by the client’s browser to display the webpage and handle interactions locally.
This contrasts with backend development, which happens on the server. The client-side approach allows for immediate user feedback and richer interactions without needing to constantly communicate back to the server for every small action, making websites feel faster and more responsive during use.
Focusing on User Interface (UI) and User Experience (UX)
Two critical concepts in frontend development are User Interface (UI) and User Experience (UX). UI refers to the visual elements users interact with: buttons, menus, layouts, typography, and colors. It’s about the look and feel, ensuring aesthetic appeal and clarity.
User Experience (UX), on the other hand, encompasses the overall feeling a user gets while interacting with the website. Is it easy to use? Is it intuitive? Does it help them achieve their goals efficiently? Good frontend development ensures both an attractive UI and a seamless UX.
For example, a well-designed UI might feature a large, clearly labeled “Add to Cart” button. Good UX ensures that clicking this button gives immediate feedback (like an animation or message) and smoothly guides the user to the next step in the purchasing process without confusion.
A Simple Analogy: Frontend as the “Interior Design” of a Website
Imagine building a house. Frontend development is like the interior design, decoration, and layout. It determines how the rooms look (colors, furniture), how easy it is to move around (layout), and how functional appliances are (interactivity like light switches).
In this analogy, the backend would be the house’s foundation, structural beams, plumbing, and electrical wiring – essential infrastructure hidden from view but crucial for the house to function. The frontend focuses purely on making the house livable, comfortable, and visually appealing for the inhabitants (users).
Just as interior design makes a house feel like a home, frontend development makes a website engaging and effective for its visitors. It’s the bridge between the underlying technology and the human user, making digital interaction possible and pleasant.
The Building Blocks: Core Frontend Technologies
Frontend development primarily relies on three foundational technologies that work together seamlessly. These are HTML (HyperText Markup Language), CSS (Cascading Style Sheets), and JavaScript (JS). Virtually every website you visit uses this core trio to structure, style, and add interactivity.
Understanding the role of each technology is fundamental to grasping frontend development. They are the essential toolkit every frontend developer uses daily to build the web experiences we encounter online. Let’s explore what each one does specifically.
HTML: Structuring the Content
HTML (HyperText Markup Language) provides the basic structure and semantic meaning of web content. It uses tags (like <p>
, <h1>
, <img>
) to define different types of content, such as paragraphs, headings, images, lists, and links. Think of HTML as the skeleton of a webpage.
For instance, an HTML tag like <h1>My Page Title</h1>
tells the browser “This text is the main heading of the page.” Similarly, <p>This is a paragraph of text.</p>
defines a standard paragraph. HTML organizes the raw content logically.
It doesn’t control how the content looks visually (that’s CSS’s job) or how it behaves interactively (that’s JavaScript’s job). HTML strictly defines the structure and the meaning of each piece of content on the page, providing the essential foundation.
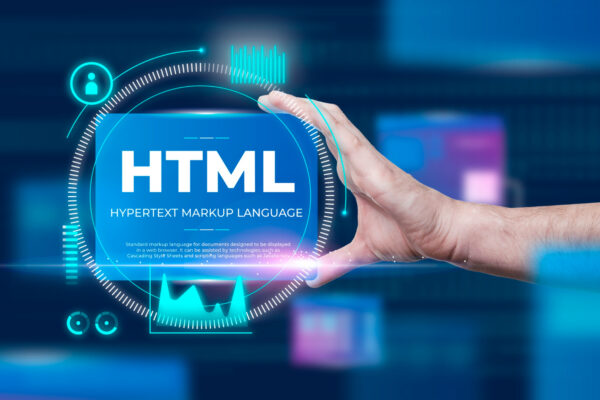
Here’s a very simple HTML example:
<!DOCTYPE html>
<html>
<head>
<title>My Simple Page</title>
</head>
<body>
<h1>Welcome!</h1>
<p>This is a basic example.</p>
<button>Click Me</button>
</body>
</html>
This code defines a page title, a main heading, a paragraph, and a button element.
CSS: Styling the Appearance
CSS (Cascading Style Sheets) is used to control the presentation, styling, and layout of the HTML content. It dictates how elements should look, including their colors, fonts, sizes, spacing, positioning, and background images. CSS is like the clothing and visual style applied to the HTML skeleton.
Using CSS, you can take the raw HTML structure and make it visually appealing. For example, you could specify that all <h1>
headings should be blue and centered, or that buttons should have rounded corners and change color when hovered over with the mouse.
CSS also plays a crucial role in responsive web design. This means ensuring the website layout adapts gracefully to different screen sizes, from large desktop monitors to small smartphone screens. This adaptability is essential for reaching users on all devices effectively.
Here’s a simple CSS example targeting the previous HTML:
body {
font-family: sans-serif;
}
h1 {
color: navy;
text-align: center;
}
button {
background-color: lightblue;
padding: 10px 20px;
border: none;
border-radius: 5px;
}
This CSS code sets a font, styles the heading, and gives the button a specific look.
JavaScript: Adding Interactivity and Behavior
JavaScript (JS) is a programming language that adds interactivity and dynamic behavior to websites. While HTML provides structure and CSS handles visuals, JavaScript makes the page react to user actions, update content dynamically without reloading, and perform complex tasks within the browser.
Common examples of JavaScript in action include interactive forms that validate input instantly, image sliders or carousels, drop-down menus that appear on click, real-time content updates (like social media feeds), interactive maps, and complex web applications like online document editors or games.
JavaScript manipulates the HTML structure (via the Document Object Model or DOM, a programming interface for HTML documents) and CSS styles in response to events like clicks, mouse movements, or keyboard input. It essentially brings the static structure and style to life.
For example, JavaScript could be used to make the previous button display a message when clicked:
// Find the button element in the HTML
const myButton = document.querySelector('button');
// Add an event listener for clicks
myButton.addEventListener('click', () => {
alert('Button was clicked!');
});
This script makes the button interactive, demonstrating JS’s role.
How HTML, CSS, and JavaScript Work Together?
These three core technologies are designed to collaborate closely. HTML establishes the content structure, CSS defines the visual presentation of that structure, and JavaScript controls the dynamic behavior and user interaction with both the structure and presentation. They form a powerful trio.
Imagine a light switch on a wall. HTML defines it as a ‘switch’ element. CSS positions it on the wall and determines its color and shape. JavaScript handles the action: when you flip the switch (user interaction), it turns the light (another element) on or off (dynamic behavior).
Modern frontend development relies heavily on the synergy between these three. Developers use them together to create the rich, interactive, and visually engaging web experiences that users expect today, ensuring both form and function are addressed effectively.
Frontend vs. Backend: What’s the Difference?
Understanding the distinction between frontend and backend development is crucial. They represent two sides of the same coin in web application development, often referred to as the client-side (frontend) and the server-side (backend). They handle different responsibilities but must work together.
Think back to the house analogy: frontend is the visible interior design, while backend is the hidden infrastructure (foundation, plumbing). Or consider a restaurant: the frontend is the dining area, menu, and waiter (what the customer interacts with), while the backend is the kitchen (where food is prepared).
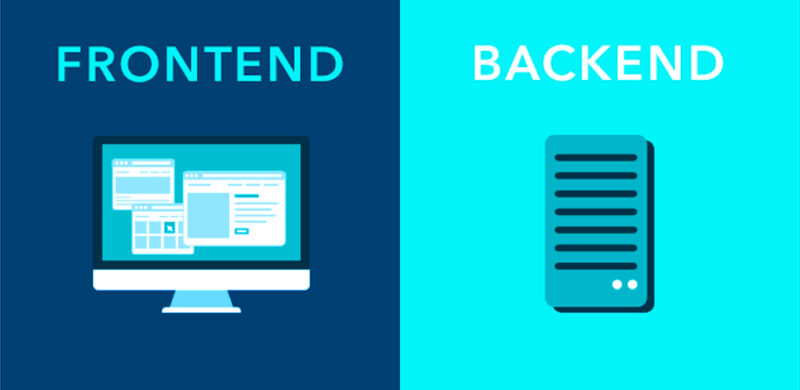
Understanding the Client-Side
As discussed, the frontend (client-side) encompasses everything the user directly interacts with in their browser. Its primary concerns are visual presentation (UI), user experience (UX), responsiveness across devices, and handling immediate user interactions using HTML, CSS, and JavaScript.
Frontend code is executed on the user’s device. Its performance depends on the user’s browser capabilities and device power. Frontend developers focus on translating designs into interactive interfaces, ensuring accessibility, and optimizing the user-facing aspects for speed and usability.
Understanding the Server-Side
The backend (server-side) refers to the part of the application that runs on the web server, hidden from the user. It handles the core logic, database interactions, user authentication, server configuration, and provides the data and services needed by the frontend.
Backend development involves using server-side programming languages (like Python, Java, Ruby, Node.js, PHP), managing databases (like MySQL, PostgreSQL, MongoDB), and building APIs (Application Programming Interfaces) to communicate with the frontend. It’s the engine that powers the application.
For example, when you log into a website, the frontend displays the login form. When you submit your credentials, the frontend sends them to the backend. The backend verifies your details against a database, manages your session, and tells the frontend whether the login was successful.
How They Communicate
Frontend and backend systems need a way to talk to each other. This communication typically happens through an API (Application Programming Interface). An API acts like a contract or a menu defining how the two systems can exchange information or request actions.
Think of the restaurant analogy again. The menu (API) lists the available dishes (data/functions). The customer (frontend) tells the waiter (API call) what they want. The waiter takes the order to the kitchen (backend), which prepares the dish and gives it back to the waiter to serve the customer.
Frontend developers use JavaScript (often with techniques like AJAX or the Fetch API) to make requests to the backend API. They ask for data (e.g., user profile information, product listings) or tell the backend to perform an action (e.g., save a user’s post, process a payment). The backend responds accordingly.
What Does a Frontend Developer Do?
A Frontend Developer is a specialized type of web developer who focuses on building and implementing the user interface and user experience components of websites and web applications. They bridge the gap between visual design and technical implementation, ensuring the user-facing side works well.
They primarily use the core technologies – HTML, CSS, and JavaScript – along with various tools and frameworks to bring designs to life. Their goal is to create interfaces that are not only visually appealing but also functional, accessible, performant, and easy for users to navigate.
Key Responsibilities and Daily Tasks
The day-to-day responsibilities of a frontend developer often include:
- Collaborating with UI/UX designers to understand design requirements and feasibility.
- Writing well-structured, semantic HTML to build the page layout.
- Applying CSS to style elements according to design specifications and ensure responsiveness.
- Implementing interactivity and dynamic features using JavaScript.
- Working with frontend frameworks or libraries (like React, Angular, Vue) to build complex applications efficiently.
- Testing code across different browsers and devices to ensure compatibility (cross-browser testing).
- Debugging issues found during testing or reported by users.
- Optimizing website performance for faster load times (e.g., optimizing images, minifying code).
- Ensuring web accessibility standards are met for users with disabilities.
- Using version control systems like Git to manage code changes and collaborate with teams.
- Communicating with backend developers to integrate frontend components with server-side logic via APIs.
- Staying updated with the latest frontend technologies, trends, and best practices in the rapidly evolving web development field.
Essential Skills for Frontend Development
To succeed as a frontend developer, a combination of technical and soft skills is necessary. Core technical skills include strong proficiency in HTML, CSS, and JavaScript. Familiarity with frontend frameworks/libraries is often required for many roles.
Understanding responsive design principles, cross-browser compatibility issues, version control (Git), and browser developer tools for debugging is essential. Knowledge of web performance optimization techniques and web accessibility (WCAG) standards is also highly valued in the industry.
Beyond technical skills, problem-solving abilities are crucial for debugging code and finding solutions. Good communication and collaboration skills are needed to work effectively with designers, backend developers, and other team members. Attention to detail ensures high-quality, pixel-perfect implementation of designs.
Tools of the Trade: Beyond the Core Three
While HTML, CSS, and JavaScript are the fundamental building blocks, the modern frontend development landscape includes a vast ecosystem of tools, frameworks, and libraries designed to make development faster, more efficient, and more powerful. Developers rarely work only with the core three anymore.
These tools help manage complexity, promote code reusability, automate repetitive tasks, and leverage community-developed solutions for common problems. Understanding these tools, at least conceptually, provides a fuller picture of contemporary frontend development practices.
A Quick Look at Frameworks and Libraries
JavaScript frameworks and libraries are collections of pre-written code that provide structure and ready-made components for building applications. They simplify common tasks and enforce development patterns. Popular examples include:
- React: A library (often called a framework) developed by Facebook for building user interfaces, known for its component-based architecture.
- Angular: A comprehensive framework developed by Google for building large-scale, complex applications.
- Vue.js: A progressive framework known for its approachability and flexibility, often considered easier to learn initially.
- jQuery: An older, widely-used library that simplified DOM manipulation and event handling, though less central in modern framework-based development.
These tools allow developers to build sophisticated single-page applications (SPAs) and dynamic interfaces more rapidly than using plain JavaScript alone. Choosing a framework often depends on project requirements, team familiarity, and performance needs. Statistics from developer surveys like the Stack Overflow Developer Survey consistently show high usage and interest in frameworks like React, Angular, and Vue.
Other Helpful Tools
Beyond frameworks, frontend developers utilize various other tools:
- Version Control Systems (VCS): Tools like Git (often used with platforms like GitHub or GitLab) are essential for tracking changes to code, collaborating with teams, and reverting to previous versions if needed.
- Package Managers: npm (Node Package Manager) and Yarn are used to install, manage, and update project dependencies (the external libraries and frameworks the project relies on).
- Build Tools & Bundlers: Tools like Webpack, Vite, or Parcel automate tasks like compiling code (e.g., converting Sass to CSS, modern JS to older compatible versions), bundling multiple files into fewer ones for better performance, and optimizing assets.
- CSS Preprocessors: Sass and Less extend CSS with features like variables, nesting, and functions, making stylesheets more maintainable and organized. The build tool typically compiles these into standard CSS.
- Code Editors: Specialized text editors like Visual Studio Code (VS Code), Sublime Text, or Atom provide features like syntax highlighting, code completion, and debugging integration, significantly improving developer productivity.
- Browser Developer Tools: Every modern browser includes powerful built-in tools for inspecting HTML elements, debugging JavaScript, analyzing network requests, and profiling performance. These are indispensable for frontend work.
Why is Frontend Development So Important?
Frontend development might seem like it’s just about making things look pretty, but its impact goes far deeper. It’s a critical aspect of any digital product because it directly influences how users perceive and interact with a business or service online. Good frontend development is essential for success.
In today’s digital-first world, a website or application often serves as the primary point of contact between an organization and its audience. The quality of the frontend experience can significantly affect user engagement, conversions, brand reputation, and overall business outcomes.
Direct Impact on User Experience and Satisfaction
The frontend is the user experience for most practical purposes. A slow, confusing, or visually unappealing interface will frustrate users, potentially causing them to leave and seek alternatives. Users expect websites to be fast, intuitive, and enjoyable to use.
Conversely, a well-crafted frontend provides a smooth, seamless experience that helps users achieve their goals efficiently. This leads to higher user satisfaction, increased engagement time, and a greater likelihood of users returning or completing desired actions (like making a purchase or signing up).
The Role in Business Success and Brand Perception
A professional, polished frontend enhances brand credibility and trustworthiness. Your website is often the first impression potential customers have of your business. A poor frontend can make even a great product or service seem unreliable or unprofessional.
Furthermore, the frontend directly impacts conversion rates. Clear navigation, compelling calls-to-action (CTAs), and a smooth checkout process (for e-commerce) are all frontend concerns that heavily influence whether a visitor becomes a customer. Optimizing the frontend is optimizing the path to business goals.
Accessibility and Performance Really Matter
Web accessibility (often abbreviated as a11y) ensures that people with disabilities can perceive, understand, navigate, and interact with websites. This is not only an ethical consideration but also a legal requirement in many regions. Frontend developers play a key role in implementing accessible HTML and interactions.
Performance is another critical factor. Studies consistently show that users abandon websites that take too long to load. Google also uses page speed as a ranking factor in search results. Frontend optimization techniques directly impact load times and responsiveness, affecting both user experience and SEO.
Getting Started with Frontend Development
We’ve journeyed through the core concepts of frontend development, exploring what it is, the essential technologies involved (HTML, CSS, JavaScript), how it differs from backend development, the role of a frontend developer, and the tools they use. We’ve also seen why it’s so crucial for modern web experiences.
Frontend development is a dynamic and rewarding field that combines creativity with technical skill. It’s about building the digital interfaces that connect people to information and services across the globe, shaping how we interact with technology every day.
Quick Recap: What We’ve Learned
- Frontend Development: Creates the user-facing, interactive part of websites/apps running in the browser (client-side).
- Core Technologies: HTML (structure), CSS (style), JavaScript (interactivity).
- Frontend vs. Backend: Frontend is what you see; backend is the hidden engine. They communicate via APIs.
- Key Focus: User Interface (UI) and User Experience (UX), performance, responsiveness, accessibility.
- Importance: Crucial for user satisfaction, business success, brand perception, and accessibility.
Interested in Learning More?
If this introduction has sparked your interest, the best way to learn frontend development is by doing! Start with the basics: learn HTML structure, then move on to CSS styling, and finally dive into JavaScript for interactivity. Many excellent free and paid resources are available online.
Consider online learning platforms like freeCodeCamp, Codecademy, MDN Web Docs (Mozilla Developer Network), or Udemy. Build small projects, experiment with code, and gradually tackle more complex challenges. The frontend development community is vast and welcoming, so don’t hesitate to explore and connect! Good luck on your learning journey.