Have you ever encountered instructions asking you to “open a shell” or “use the command line”? Perhaps you’ve seen programmers in movies typing cryptic commands onto a black screen. This interface, often intimidating at first glance, is a powerful tool called a shell, and understanding it is fundamental in computing.
A shell is essentially a program that acts as an interpreter between you and the operating system’s core (the kernel). It takes your typed text commands and translates them into actions for the computer to perform. Think of it as your direct line to controlling your system’s functions efficiently.
This guide aims to demystify the shell completely. We will explore precisely what it is, why it’s incredibly useful, how it differs from related terms like “terminal,” and the various types you might encounter. We’ll make this journey approachable, even if you’re entirely new to the command line.
Understanding the shell isn’t just for seasoned system administrators or developers anymore. In an increasingly automated world, knowing your way around a command line interface, or CLI, provides significant advantages for efficiency, control, and understanding how computers truly operate beneath the graphical surface you usually interact with daily.
Defining the Shell: Your Computer’s Interpreter
So, what exactly is a shell in the world of computers? A shell is a special user interface program that interprets command lines (text commands) entered by a user or from a file (a script), and then executes them by instructing the operating system. It’s the bridge facilitating your conversation with the OS.
Imagine you need to communicate with someone who speaks a different language. You might use a human interpreter. Similarly, the shell acts as this interpreter between your human-readable commands and the complex language understood by the operating system’s core component, known as the kernel.
The kernel is the heart of the operating system, managing hardware and core system functions. The shell provides you, the user, with access to these kernel functions through commands, shielding you from the kernel’s intricate details while enabling powerful interaction and control over the system.
Most shells operate via a Command Line Interface (CLI). This means you interact by typing commands rather than clicking icons in a Graphical User Interface (GUI). While GUIs are user-friendly for many tasks, CLIs often offer more direct control, speed, and automation capabilities for specific operations.
Think of the difference between ordering coffee by telling the barista exactly what you want (CLI) versus pointing at pictures on a menu (GUI). Both achieve the goal, but the direct conversation allows for more precise customization and efficiency once you know the language (the commands).
Therefore, the shell is fundamentally a command processor. It reads your input, figures out what you intend based on the command and its arguments, and then makes the operating system carry out that task, displaying any results back to you on the screen.
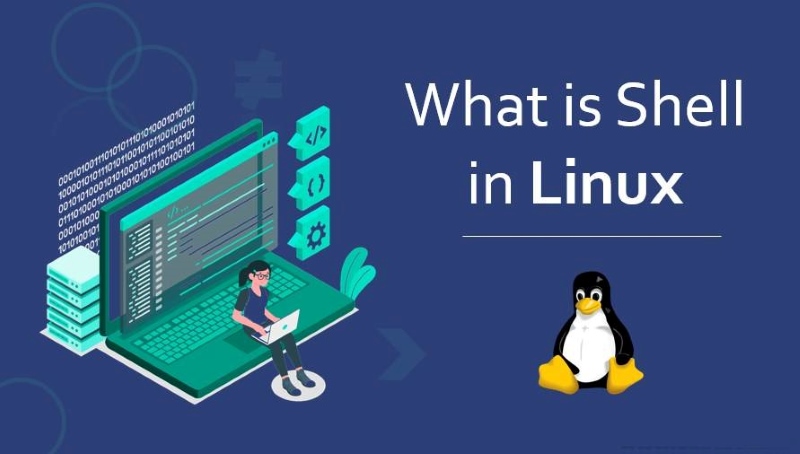
The Purpose and Power of the Shell
Why do we even need shells when graphical interfaces are so common? The shell serves several critical purposes, making it an indispensable tool in various computing domains, from personal use to large-scale server management and software development. Its power lies in its directness and efficiency.
Direct Operating System Communication
One primary purpose is providing direct, unfiltered access to the operating system’s capabilities. Unlike GUIs, which might abstract or limit certain functions, a shell often allows you to interact with nearly every aspect of the OS, offering finer control over system behavior, configuration, and resources.
This direct line to the kernel is crucial for tasks requiring precise system manipulation. For instance, managing network configurations, adjusting system parameters, or diagnosing complex issues often necessitates using shell commands that might not have a graphical equivalent or offer the same level of detail.
Efficiency for Complex or Repetitive Tasks
For users familiar with its commands, the shell can be significantly faster than navigating multiple GUI windows and menus. Typing a single command, like mv *.log /backup/logs/
, can rename and move numerous files instantly, a task potentially requiring many clicks and drags graphically.
This efficiency compounds dramatically when dealing with repetitive actions. Instead of manually performing the same sequence of clicks hundreds of times, you can use the shell to execute the task with one command or, even better, automate it entirely using a simple script.
Automation Through Scripting
This leads to one of the shell’s most powerful features: shell scripting. You can write sequences of shell commands into a file (a script) and then execute that file. The shell reads the commands sequentially, automating complex workflows, administrative routines, or any repetitive task imaginable.
Imagine needing to back up specific files every night, process web server logs for analysis, or set up a consistent development environment on multiple machines. Shell scripts make these tasks reliable and automatic, saving countless hours and reducing the potential for human error in manual processes.
Essential System Administration Tool
For system administrators (sysadmins) managing servers, networks, and infrastructure, the shell is not just useful; it’s often the primary interface. Many servers, especially Linux-based ones common in web hosting and cloud computing, operate without a GUI (“headless”) to conserve resources, making shell access essential.
Sysadmins rely on shell commands for installing and updating software, managing user accounts and permissions, monitoring system performance (using commands like top
or htop
), configuring security settings (like firewalls), troubleshooting problems, and managing services like web servers (Apache, Nginx) or databases.
Critical for Server Management
Relatedly, managing web servers, cloud instances (like AWS EC2 or Google Compute Engine), or Virtual Private Servers (VPS) typically involves connecting remotely via protocols like SSH (Secure Shell) and interacting directly with the server’s shell. This is standard practice for deployment and maintenance.
Tasks like deploying web applications, restarting services, checking server logs for errors, managing databases via command-line tools (like mysql
or psql
), or configuring web server settings are all commonly performed through the server’s shell interface, offering direct control over the hosting environment.
Indispensable in Development Workflows
Modern software development heavily relies on shell commands. Developers use the shell constantly for tasks like version control (using Git commands like git clone
, git commit
), managing software dependencies (using package managers like npm
, pip
, apt
, yum
), compiling code, running tests, and deploying applications.
Integrated Development Environments (IDEs) often embed terminals, but understanding the underlying shell commands provides greater flexibility and control. Many build systems, containerization tools (like Docker), and orchestration platforms (like Kubernetes) are primarily driven through their respective CLIs within a shell.
Behind the Scenes: How a Shell Processes Commands
Understanding how a shell works transforms it from a mysterious black box into a logical tool. When you type a command and press Enter, a sequence of steps occurs very quickly to translate your request into action. Let’s break down this fundamental process.
First, the shell displays a prompt, often a symbol like $
or #
(the #
usually indicates administrative privileges, often called the “root” user). This prompt signals the shell is ready to accept your input. You then type your command, like ls -l Documents
.
When you press Enter, the terminal emulator (the window program you’re likely using) sends this line of text to the shell program running inside it. The shell then reads this input line: ls -l Documents
. It needs to figure out what this means.
The shell performs parsing. It breaks the input line into words or tokens, typically separated by spaces. In our example, it sees “ls”, “-l”, and “Documents”. The first word, ls
, is identified as the command or program name to be executed.
The subsequent words, -l
and Documents
, are treated as arguments or options passed to the ls
command. These modify the command’s behavior (-l
asks for a detailed listing format) or specify what the command should operate on (Documents
indicates the target directory).
Next, the shell needs to find the ls
program. It checks if ls
is a built-in command (handled directly by the shell itself). If not, it searches for an executable file named ls
in a list of directories defined by an environment variable called PATH
.
Environment variables are named values stored by the shell that affect how it and other programs behave. The PATH
variable contains a colon-separated list of directories (like /bin
, /usr/bin
) where the shell looks for executable programs. This is how it finds /bin/ls
.
Once the program (/bin/ls
in this case) is located, the shell requests the operating system kernel to create a new process and run this program. It also passes the arguments (-l
, Documents
) to the new process. Control is temporarily handed over to the ls
program.
The ls
program runs, performs its task (listing files in the Documents directory in long format), and generates output. This output is typically sent to a channel called standard output (stdout). Error messages usually go to standard error (stderr). Your keyboard input goes to standard input (stdin).
Finally, the shell captures the output from stdout
(and potentially stderr
) generated by the ls
command. It then displays this output back to you in the terminal window. Once the ls
command finishes, the shell displays its prompt again, ready for your next instruction.
Shell vs. Terminal Emulator: What’s the Difference?
A very common point of confusion for newcomers is the distinction between a “shell” and a “terminal” (or “terminal emulator”). While often used together, they are distinct components performing different roles. Understanding this difference clarifies how you interact with the command line.
A terminal emulator is the graphical application window that provides the text-based interface on your screen. Think of programs like Gnome Terminal, Konsole on Linux, Terminal.app or iTerm2 on macOS, or Windows Terminal and the older Command Prompt window on Windows. It emulates the old physical computer terminals.
These emulator programs handle displaying the text characters, processing keyboard input, managing colors, fonts, and window size, and essentially providing the visual container. The terminal emulator itself doesn’t understand or execute your commands like ls
or cd
. Its job is presentation and basic input/output handling.
The shell, on the other hand, is the command processor program running inside the terminal emulator window. It’s the “brain” that actually interprets the commands you type into the terminal, interacts with the operating system kernel to execute them, and sends the results back to the terminal for display.
Think of a restaurant analogy: The terminal emulator is like the physical table, menu, and perhaps the screen displaying your order number. It’s the interface you see and interact with directly. It presents information and takes your raw input (your order written down).
The shell is like the waiter or the Point-of-Sale system that takes your specific order (the command), understands what “cheeseburger, no onions” means (parses the command and arguments), communicates it correctly to the kitchen (the kernel), and brings back the food (the command’s output) to your table (the terminal).
So, when you “open a terminal,” you are starting the terminal emulator application. This application, in turn, usually starts a default shell program (like Bash or Zsh) running within it, ready to accept your commands. You type into the terminal, but the shell does the processing.
Historically, terminals were physical hardware devices – bulky monitors with keyboards connected to a central mainframe computer. Today’s terminal emulators are software programs that replicate the behavior of these classic terminals within our modern graphical desktop environments, providing the necessary window for the shell to operate in.
Exploring Different Shells: Types and Examples
Just as there are different web browsers (Chrome, Firefox, Safari), there are various shell programs available, each with its own history, features, syntax variations, and typical use cases. While they share the core purpose of interpreting commands, their specific capabilities can differ significantly.
Unix/Linux Shells
The Unix and Linux world boasts a rich history of shell development, largely stemming from two main families: the Bourne shell family and the C shell family. Most modern Linux distributions and macOS use shells derived from the Bourne lineage due to their powerful scripting features.
- sh (Bourne Shell): Developed by Stephen Bourne at Bell Labs in the late 1970s, this was one of the earliest and most influential shells. While not often used interactively today, its syntax forms the basis for many successors and basic system scripts (
/bin/sh
often links to a more modern shell like Bash running in compatibility mode). - Bash (Bourne Again SHell): Created by Brian Fox for the GNU Project, Bash is arguably the most widely used shell, especially on Linux systems where it’s often the default login shell. It extends the original Bourne shell with features like command history, tab completion, better scripting capabilities, and job control. Its ubiquity makes Bash scripting highly portable across Unix-like systems. [Bash is estimated to be the default shell for over 70% of Linux systems – specific statistic source needed].
- Zsh (Z Shell): Developed by Paul Falstad, Zsh is another powerful Bourne-style shell that has gained immense popularity, particularly among developers. It incorporates features from Bash, Ksh, and Tcsh, adding enhancements like improved autocompletion, sophisticated globbing (wildcard matching), spelling correction, themes, and extensive plugin support (e.g., via frameworks like “Oh My Zsh”). Zsh became the default shell in macOS starting with Catalina (10.15).
- Ksh (KornShell): Designed by David Korn at Bell Labs, Ksh aimed to combine the scripting strengths of the Bourne shell with the interactive features of the C shell. It introduced associative arrays and improved arithmetic evaluation. While less common as a default interactive shell now, it remains influential and used in specific enterprise environments.
- Csh (C Shell): Created by Bill Joy at UC Berkeley, Csh introduced features geared towards interactive use, such as command history and aliasing, with a syntax deliberately modeled after the C programming language. However, its scripting capabilities are often considered less robust or predictable than Bourne-style shells, leading to less usage for complex automation. Tcsh is an enhanced version.
- Fish (Friendly Interactive SHell): A more modern shell designed with a strong focus on user-friendliness and out-of-the-box usability. Fish features syntax highlighting, extensive autocompletions based on history and context, and simpler scripting syntax, aiming to be easy to learn and use without complex configuration, though its syntax differs from traditional Bourne shells.
Windows Shells
Microsoft Windows has its own history of command-line interpreters, evolving significantly over time to meet modern administrative and automation needs, integrating tightly with the Windows ecosystem.
- Command Prompt (cmd.exe): The traditional command-line interpreter inherited from MS-DOS and present in all modern Windows versions. It’s suitable for basic file operations, running batch scripts (.bat, .cmd), and simple administrative tasks. However, its scripting language is limited, and it lacks many features found in Unix shells like robust pipelines.
- PowerShell: Introduced by Microsoft as a much more powerful replacement for Command Prompt. PowerShell is an object-oriented automation engine and scripting language built on the .NET framework. It uses “cmdlets” (command-lets) that output objects, not just text, allowing for complex data manipulation through pipelines. It offers features like remoting, desired state configuration, and access to WMI/CIM for deep system management. Initially Windows-only, PowerShell is now cross-platform (Windows, Linux, macOS).
Choosing a shell often depends on the operating system, personal preference, and specific needs. For general Linux/macOS use, Bash or Zsh are excellent choices. For modern Windows administration and automation, PowerShell is the standard. Understanding the basics, however, translates well across most shells.
Key Functions: What Can a Shell Do?
Beyond just running programs, shells provide built-in capabilities and work with external utilities to perform a wide range of essential tasks. Mastering these core functions unlocks the true power of the command line for managing your system and files efficiently.
Executing Commands and Programs
The most fundamental function is running commands. This includes built-in shell commands (like cd
, pwd
, echo
), external utility programs provided by the OS (like ls
, grep
, find
), and any other application or script installed on the system. Simply type the command name and press Enter.
Example: To see the current date and time, you run the date
utility. date
Example: To run a Python script named myscript.py
. python myscript.py
Navigating the File System
Shells allow you to move around the directory structure (folders) of your computer. Key commands include:
pwd
(print working directory): Shows your current location.cd
(change directory): Moves you to a different directory.cd ..
moves up one level.cd /home/user/Documents
moves to a specific path.cd ~
often goes to your home directory.ls
(list directory contents): Shows files and folders in the current or specified directory.
Example: Navigate to your home directory’s Documents folder and list its contents. cd ~/Documents
pwd
ls
Managing Files and Directories
You can create, delete, copy, move, and rename files and directories directly from the shell:
mkdir
(make directory): Creates a new directory.mkdir my_project
rmdir
(remove directory): Deletes an empty directory.rmdir old_stuff
touch
(update timestamp / create file): Creates an empty file if it doesn’t exist.touch new_file.txt
cp
(copy): Copies files or directories.cp source.txt destination.txt
orcp report.docx /backups/
mv
(move/rename): Moves files/directories or renames them.mv old_name.txt new_name.txt
ormv file.log /var/log/archive/
rm
(remove): Deletes files (use with caution!).rm temporary_file.tmp
. The-r
flag is needed to remove directories and their contents:rm -r unwanted_folder
(again, be careful!).
Example: Create a directory, create a file inside it, then copy that file. mkdir project_alpha
cd project_alpha
touch main_script.py
cp main_script.py main_script_backup.py
ls
Managing Processes
Shells let you view and control running programs (processes):
ps
(process status): Lists currently running processes. Often used with flags likeps aux
(on Linux/macOS) to see all processes with details.top
orhtop
: Display dynamic, real-time information about running processes and system resource usage (CPU, memory).kill
: Sends a signal to a process, typically to terminate it. You need the Process ID (PID).kill 12345
sends a termination signal.kill -9 12345
sends a forceful kill signal.- Background execution (
&
): Run a command in the background so the shell prompt returns immediately.long_running_script.sh &
- Job control (
jobs
,fg
,bg
): Manage background processes started from the current shell.
Example: Find the process ID (PID) of a running ‘notepad’ process and terminate it (conceptual example). ps aux | grep notepad
(Find the PID, e.g., 5678) kill 5678
Handling Input/Output (Redirection)
Shells allow you to control where a command gets its input from and where its output goes:
>
(output redirection): Sends standard output (stdout) to a file, overwriting the file if it exists.ls -l > file_list.txt
>>
(append output): Appends stdout to a file.echo "Log entry" >> activity.log
<
(input redirection): Takes standard input (stdin) from a file instead of the keyboard.sort < unsorted_names.txt
2>
(error redirection): Redirects standard error (stderr) to a file.run_script.sh 2> error_log.txt
Example: List files and save the list to listing.txt
, then append an error message from a potentially failing command to errors.log
. ls > listing.txt
failing_command 2>> errors.log
Connecting Commands (Pipes)
The pipe |
symbol is incredibly powerful. It takes the standard output (stdout) of the command on the left and sends it directly as standard input (stdin) to the command on the right, chaining them together.
Example: List all files (ls -l
), filter to show only lines containing “.txt” (grep .txt
), and then count how many lines there are (wc -l
). ls -l | grep .txt | wc -l
This chaining allows you to build complex data processing workflows from simple, single-purpose command-line utilities, embodying the Unix philosophy of “do one thing and do it well.”
Introduction to Shell Scripting: Automating Tasks
One of the most compelling reasons to learn the shell is shell scripting. This involves writing a series of shell commands into a plain text file. You can then instruct the shell to execute this file, running all the commands sequentially, effectively automating a task or workflow.
Think of a script as a recipe the shell follows. Instead of typing each command manually every time, you write them down once in the script file. This is invaluable for tasks that are repetitive, complex, or need to be performed consistently without human intervention.
Shell scripts typically start with a shebang line, like #!/bin/bash
or #!/usr/bin/env python
. This line tells the operating system which interpreter (which shell or program) should be used to execute the commands within the script file. For Bash scripts, #!/bin/bash
is common.
Consider a simple backup task. You might manually run commands like mkdir /backup/$(date +%Y-%m-%d)
to create a dated backup folder, then cp -r /home/user/Documents /backup/$(date +%Y-%m-%d)/
to copy your documents. This requires typing each time.
A basic Bash script (backup_docs.sh
) could automate this:
#!/bin/bash
# Simple script to backup documents to a dated folder
BACKUP_DIR="/backup/$(date +%Y-%m-%d)"
SOURCE_DIR="/home/user/Documents"
echo "Creating backup directory: $BACKUP_DIR"
mkdir -p "$BACKUP_DIR" # -p creates parent dirs if needed
echo "Copying files from $SOURCE_DIR..."
cp -r "$SOURCE_DIR" "$BACKUP_DIR/"
echo "Backup complete!"
You would make the script executable (chmod +x backup_docs.sh
) and then run it simply with ./backup_docs.sh
. The shell reads the file and executes each command.
The possibilities are vast: automating software builds and deployments, processing log files for reporting, managing system configurations, running scheduled tasks (via tools like cron
), setting up development environments, and much more. Shell scripting is a cornerstone of automation in IT operations (DevOps) and system administration.
While this guide focuses on what a shell is, understanding its role as a scripting engine is crucial. It transforms the shell from a simple command executor into a powerful programming environment for controlling and automating your operating system and tasks effectively.
Why Understanding the Shell Matters
We’ve journeyed through the definition, purpose, mechanics, and variations of the computer shell. From its role as a command interpreter to its power in automation via scripting, the shell remains a fundamental and highly relevant component of modern computing, far from being just a relic of the past.
Understanding the shell means gaining deeper insight into how your operating system works. It equips you with the ability to perform tasks more efficiently, automate repetitive workflows, and manage systems (whether your personal computer, a web server, or cloud infrastructure) with greater precision and control than often possible through graphical interfaces alone.
While GUIs provide accessibility, the Command Line Interface offered by shells provides unparalleled power and flexibility. It’s an essential skill for system administrators, developers, DevOps engineers, data scientists, and even power users who want to optimize their interaction with their digital environment. The concepts learned apply broadly across different shells and operating systems.
Don’t be intimidated by the text-based interface. Start simple, learn basic commands for navigation and file management, and gradually explore more advanced concepts like redirection, pipes, and scripting as needed. The investment in learning the shell pays significant dividends in efficiency and capability. It’s truly an essential tool in the modern tech landscape.
Shell FAQs
Let’s address a few common questions that often arise when learning about shells:
Q: Is the command prompt (CMD) a shell? A: Yes, absolutely. Command Prompt (cmd.exe) is the default command-line interpreter and shell for older versions of Windows and remains available in current versions. While less powerful than modern alternatives like PowerShell or Unix shells like Bash, it fulfills the core role of interpreting and executing commands.
Q: Is Bash the only shell for Linux? A: No, definitely not. While Bash (Bourne Again SHell) is the default shell on many popular Linux distributions (like Ubuntu, Fedora before version 33), Linux supports a wide variety of shells. Users can easily install and switch to others like Zsh, Fish, Ksh, or Csh based on their preferences.
Q: Do I need to use the shell if I have a GUI? A: Need? Not always for basic tasks. But using the shell offers significant advantages in efficiency, automation, control, and access to specific tools, especially for development, server management, or complex system administration. Many powerful operations are faster or only possible via the shell.
Q: What is ‘shell access’ in web hosting? A: Shell access, often provided via SSH (Secure Shell), means your hosting provider allows you to log directly into the server’s command-line environment (its shell). This provides direct control to manage files, install software, run scripts, and configure the server beyond what a typical web-based control panel might offer.