You’ve likely heard about JavaScript, the language powering interactive websites. But its influence now extends far beyond the browser. This guide introduces Node.js, a pivotal technology driving much of today’s web infrastructure and beyond.
Are you curious about what Node.js actually is? Perhaps you wonder how it works or why so many developers choose it. This comprehensive guide aims to answer all your questions clearly. We will explore its definition, core mechanics, benefits, and common uses in an easy-to-understand way.
Our journey will take us from basic concepts to practical applications. By the end, you’ll have a solid grasp of Node.js and its significance in the tech landscape. Let’s dive into understanding this powerful tool that has reshaped server-side development and more.
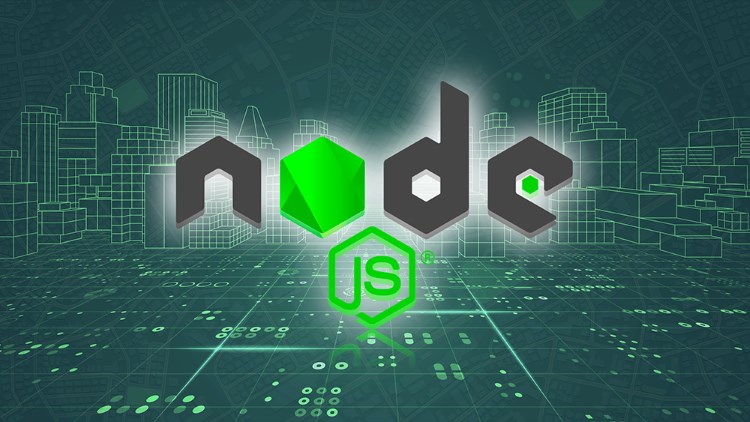
Defining Node.js: More Than Just JavaScript
The Simple Definition: Node.js Explained
Node.js is an open-source, cross-platform JavaScript runtime environment. It empowers developers to run JavaScript code outside a web browser. Built on Google’s high-performance V8 engine, it excels at creating fast, scalable server-side applications and network tools. Think of it as JavaScript unleashed.
This runtime environment provides everything needed to execute a program written in JavaScript on servers. It includes libraries and tools that extend JavaScript’s capabilities beyond simple browser scripting. This enables developers to handle tasks like file system operations, networking, and database interactions efficiently.
It’s crucial to understand its role clearly from the start. Node.js itself isn’t a new programming language you need to learn separately. If you already know JavaScript, you possess the foundational language skill needed to start working effectively within the Node.js environment.
Analogy: Understanding a Runtime Environment
Imagine JavaScript is a powerful, versatile electric drill (the programming language). Usually, you use this drill inside your home workshop (the web browser) for various tasks. The browser provides the power outlet and specific tool attachments needed for web-related jobs.
Now, picture Node.js as a specialized, portable generator and toolbox (the runtime environment). This setup allows you to take your electric drill (JavaScript) outside the home workshop. You can now use it on a construction site (a server) to build much larger structures (backend applications).
Node.js provides the necessary power (engine) and specialized tools (libraries) for JavaScript to work effectively in this new, demanding server environment. It extends JavaScript’s reach, enabling it to tackle tasks fundamentally different from manipulating web page elements inside a browser environment.
Key Takeaways: What Node.js is NOT
Let’s clear up some common points of confusion right away. Firstly, Node.js is not a programming language. It doesn’t introduce new syntax rules distinct from JavaScript. Instead, it executes standard JavaScript code, leveraging your existing knowledge of the language for server-side tasks.
Secondly, Node.js is not a web framework like Express.js, Koa, or NestJS. Frameworks are built on top of Node.js to simplify specific development tasks, like building web applications or APIs. Node.js provides the foundational runtime upon which these frameworks operate.
Finally, while widely used for web servers, Node.js isn’t limited to just that. Its capabilities extend to creating command-line tools, build automation scripts, desktop applications (with frameworks like Electron), and even Internet of Things (IoT) devices, showcasing its remarkable versatility.
How Does Node.js Work? The Core Concepts
Understanding how Node.js achieves its renowned efficiency requires looking at its core components. Its architecture is specifically designed for building applications that need to handle many connections simultaneously, such as web servers or real-time communication platforms. Let’s explore these key pieces.
Built on Chrome’s V8 Engine
At the heart of Node.js lies Google’s V8 JavaScript engine. This is the same high-performance engine that powers Google Chrome and other Chromium-based browsers. V8 doesn’t just interpret JavaScript; it compiles it directly into optimized native machine code before execution.
This compilation process significantly boosts execution speed compared to traditional interpreters. V8, primarily written in C++, constantly optimizes code during runtime. By leveraging V8, Node.js ensures that your server-side JavaScript runs incredibly fast, often rivaling code written in traditionally compiled languages.
The choice of V8 was pivotal for Node.js’s success. It provided a robust, battle-tested, and continuously improving foundation. This allows Node.js developers to benefit directly from the massive engineering effort Google invests in making JavaScript execution faster and more efficient within the Chrome browser.
The Magic of Non-Blocking I/O
To grasp Node.js’s efficiency, we must understand Input/Output (I/O) operations. These are tasks where the program needs to wait for something external, like reading data from a file, accessing a database, or receiving information over the network. These are common bottlenecks.
Traditionally, many server environments use blocking I/O. Imagine a waiter taking your order, going to the kitchen, waiting for the dish to be fully cooked, delivering it to you, and only then taking the next customer’s order. The waiter is “blocked” while waiting.
Node.js, however, primarily uses non-blocking I/O. Picture a different waiter taking your order, giving it to the kitchen, and immediately moving on to take orders from other tables. When a dish is ready, the kitchen notifies the waiter, who then delivers it.
This non-blocking approach means Node.js doesn’t waste time waiting idly for I/O tasks to complete. While one operation (like a database query) is in progress, Node.js can start handling other incoming requests or tasks. This dramatically improves throughput and efficiency, especially for applications with many simultaneous users.
Understanding the Event Loop
So, how does Node.js manage all these non-blocking operations with just a single main thread? The answer lies in the event loop. This is the core mechanism that allows Node.js to handle concurrency efficiently without relying heavily on traditional multi-threading models used by other platforms.
Think of the event loop like an attentive restaurant coordinator. It constantly checks a queue for incoming events (like new user requests or completed I/O operations). When an event arrives, the coordinator quickly delegates the task (e.g., running a specific JavaScript function or starting an I/O request).
For quick tasks (like simple calculations), the function runs directly. For longer I/O tasks (database queries, file reads), Node.js offloads them to the underlying system (often using a library called Libuv). The event loop doesn’t wait; it immediately moves to the next event in the queue.
When an offloaded I/O task finishes, the system places a corresponding “completion event” back into the queue. The event loop eventually picks up this completion event and executes the associated callback function (or resolves a Promise, or continues after an await
statement) – the JavaScript code designated to run after the I/O is done.
This continuous cycle—checking the queue, executing quick tasks, offloading long I/O tasks, processing completion events—is what allows a single-threaded Node.js application to handle thousands of concurrent connections efficiently. It ensures the main thread is never blocked by slow I/O operations, keeping the application responsive.
Why Use Node.js? Key Features and Benefits
Node.js didn’t become popular by accident. Its unique architecture provides several compelling advantages that make it an excellent choice for a wide range of development projects. Let’s explore the key features and benefits that attract developers and organizations worldwide.
Speed and Performance
One of the most cited benefits is raw speed. Thanks to the V8 engine compiling JavaScript to machine code and the non-blocking I/O model, Node.js applications often exhibit impressive performance. Tasks involving network requests or database interactions run very efficiently without blocking the main thread.
This performance is particularly noticeable in applications requiring real-time data exchange or handling numerous simultaneous connections. Benchmarks frequently show Node.js outperforming traditional multi-threaded platforms for specific types of I/O-bound workloads, making it ideal for modern web services.
High Scalability
Node.js applications are inherently scalable. The event-driven, non-blocking architecture allows a single Node.js instance to handle a vast number of concurrent connections with relatively low memory usage compared to thread-per-connection models. This makes scaling applications horizontally (adding more servers) very cost-effective.
This scalability is a primary reason why Node.js is favored for building microservices. These small, independent services need to handle many requests efficiently. It’s also ideal for real-time applications like chat systems or live dashboards, where maintaining numerous persistent connections is essential for functionality.
JavaScript Everywhere (Full-Stack)
A significant advantage is the ability to use JavaScript across the entire stack – both for the frontend (browser) code and the backend (server) code. This unification simplifies development workflows and allows teams to specialize in a single language, potentially improving collaboration and code reuse.
Frontend developers can more easily transition to backend work, and vice versa. Teams can share validation logic or utility functions between client and server, reducing code duplication and potential inconsistencies. This “JavaScript everywhere” paradigm streamlines the development process significantly for many organizations.
The Power of NPM (Node Package Manager)
Node.js comes bundled with NPM (Node Package Manager). NPM is both a command-line tool and the world’s largest online registry of open-source software packages (modules). As of early 2025, the registry hosts well over two million packages, providing pre-built solutions for nearly any conceivable task.
This vast ecosystem drastically accelerates development. Need to connect to a database, handle image uploads, or integrate with a third-party API? There’s almost certainly an NPM package available. Managing project dependencies is straightforward using the package.json
file, ensuring consistent builds across different environments.
NPM embodies the collaborative spirit of open-source software. Developers can easily share their own modules and leverage the work of countless others. This collective effort means you rarely have to build common functionalities from scratch, saving significant time and resources during project development.
Thriving Community & Ecosystem
Node.js benefits from a large, active, and vibrant global community. This translates into abundant resources: tutorials, blogs, forums (like Stack Overflow), courses, and extensive documentation are readily available. If you encounter a problem, chances are someone else has already solved it and shared the solution.
This active community also drives a rich ecosystem of frameworks and tools built upon Node.js. Popular web frameworks like Express.js, Koa.js, and NestJS provide robust structures for building applications faster. Numerous specialized libraries cater to specific needs, further enhancing Node.js’s capabilities.
Cross-Platform Development
Node.js is designed to be cross-platform. This means you can develop your application on one operating system (like macOS or Windows) and deploy it seamlessly on another (like Linux) without major code changes. This flexibility simplifies both development and deployment workflows significantly.
It ensures that development teams using different operating systems can collaborate effectively on the same codebase. It also provides freedom in choosing hosting environments, as Node.js runs consistently across major server platforms, supporting diverse infrastructure choices for deployment.
What is Node.js Used For? Common Applications
Given its unique strengths, Node.js has become a go-to technology for various types of applications, particularly those involving network communication and real-time interactions. Let’s explore some of the most common and effective use cases where Node.js truly shines.
- Backend APIs (RESTful APIs, GraphQL APIs): Node.js excels at building the backend Application Programming Interfaces (APIs) that power modern web and mobile applications. Its ability to handle many concurrent requests efficiently makes it perfect for serving data to clients quickly and reliably. Frameworks like Express.js streamline API creation.
- Real-Time Applications: Think chat applications, live sports updates, collaborative editing tools, or online multiplayer games. Node.js’s event-driven architecture, often combined with WebSockets (a technology for persistent two-way communication), is ideal for applications requiring instant data exchange between server and clients.
- Microservices: Organizations increasingly adopt a microservice architecture, breaking down large applications into smaller, independent services. Node.js’s low resource footprint, fast startup time, and scalability make it an excellent choice for building these individual microservices that communicate over the network.
- Single Page Applications (SPAs): While frameworks like React, Angular, or Vue handle the frontend, Node.js often powers the backend API serving data to these complex, interactive SPAs. Its synergy with JavaScript on the frontend makes it a natural fit for the server-side component.
- Streaming Applications: Node.js is proficient at handling data streams, whether for video and audio streaming services or processing large datasets chunk by chunk. Its built-in Stream API allows developers to process data efficiently without loading everything into memory at once, crucial for performance.
- Command-Line Interface (CLI) Tools: Many popular developer tools and build utilities are built using Node.js. Its ability to easily interact with the file system and execute system commands makes it convenient for creating powerful, cross-platform command-line tools to automate tasks.
- Server-Side Rendering (SSR): For performance or SEO reasons, some applications render initial web pages on the server before sending them to the browser. Node.js is often used in conjunction with frontend frameworks to perform this server-side rendering, improving initial load times and search engine visibility.
Numerous leading technology companies leverage Node.js extensively, including Netflix, LinkedIn, PayPal, Uber, and even NASA. This widespread adoption by industry leaders serves as strong evidence of its capabilities and reliability in demanding, large-scale production environments, reinforcing its credibility (E-E-A-T).
Getting Started with Node.js
Feeling inspired to try Node.js yourself? Getting started is relatively straightforward. Here’s a quick guide on installing Node.js and taking your very first steps into its world, setting you on the path to leveraging its power for your own projects.
Installation
The easiest way to install Node.js is to download the official installer from the Nodejs.org website. They provide installers for Windows, macOS, and pre-compiled Linux binaries. Choose the LTS (Long-Term Support) version for stability, as it receives bug fixes and security updates for an extended period.
However, a highly recommended best practice, especially if you work on multiple projects, is using a Node Version Manager (NVM). Tools like nvm
(for Linux/macOS) or nvm-windows
allow you to install and easily switch between different Node.js versions on the same machine without conflicts.
Your First “Hello World” (Optional Simple Example)
Once installed, you can verify it by opening your terminal or command prompt and typing node -v
. This should display the installed Node.js version. Now, let’s create a minimal Node.js script. Create a file named app.js
and add the following line:
console.log("Hello, Node.js World!");
Save the file. In your terminal, navigate to the directory where you saved app.js
and run it using the command: node app.js
. You should see the message “Hello, Node.js World!” printed to your console. Congratulations, you’ve run your first Node.js program!
Here’s a slightly more advanced example creating a basic HTTP web server:
const http = require('http'); // Import the built-in HTTP module
const hostname = '127.0.0.1'; // Localhost IP
const port = 3000; // Port number
// Create the server
const server = http.createServer((req, res) => {
res.statusCode = 200; // OK status
res.setHeader('Content-Type', 'text/plain');
res.end('Hello from Node.js Server!\n'); // Send response
});
// Start listening for requests
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Save this as server.js
and run node server.js
. Open your web browser to http://127.0.0.1:3000
, and you’ll see the message from your running Node.js server! This demonstrates Node.js’s capability to handle web requests directly.
Next Steps & Learning Resources
Your journey with Node.js is just beginning! To continue learning, explore the official Node.js documentation available at Nodejs.org – it’s comprehensive and accurate. Numerous online tutorials, courses (on platforms like Udemy, Coursera, freeCodeCamp), and blogs offer guided learning paths.
Experiment by exploring the vast NPM registry. Try installing and using different packages to add functionality to your test projects. Consider learning a popular web framework like Express.js, which provides helpful structures and utilities specifically designed for building web applications and APIs with Node.js.
Conclusion: Is Node.js Right for You?
We’ve explored the fundamentals of Node.js: it’s a powerful JavaScript runtime environment built on the V8 engine, designed for speed and scalability through its non-blocking, event-driven architecture. It allows you to use JavaScript on the server, benefiting from the huge NPM ecosystem.
Its key strengths lie in handling numerous concurrent connections efficiently, making it ideal for APIs, real-time applications, microservices, and streaming services. The ability to use JavaScript across the stack and its vibrant community further enhance its appeal for modern development teams.
If you’re building applications that require high concurrency, real-time features, or if you want to leverage your JavaScript skills for backend development, Node.js is definitely worth considering. Its performance, scalability, and vast ecosystem provide a compelling platform for building robust, modern software.
Node.js has fundamentally changed the landscape of server-side development. By understanding its core principles and strengths, you are now better equipped to decide if it’s the right tool for your next project. We encourage you to continue exploring and experimenting with this versatile technology.